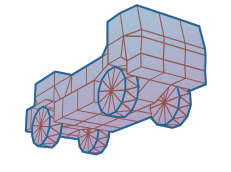 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
2 #ifndef __OGRE_SHADER_PARTICLE_RENDERER_H__
3 #define __OGRE_SHADER_PARTICLE_RENDERER_H__
6 #include <OgreParticleSystemRenderer.h>
7 #include <OgreVector2.h>
73 String doGet(
const void* target)
const;
74 void doSet(
void* target,
const String& val);
81 String doGet(
const void* target)
const;
82 void doSet(
void* target,
const String& val);
89 String doGet(
const void* target)
const;
90 void doSet(
void* target,
const String& val);
97 String doGet(
const void* target)
const;
98 void doSet(
void* target,
const String& val);
105 String doGet(
const void* target)
const;
106 void doSet(
void* target,
const String& val);
113 String doGet(
const void* target)
const;
114 void doSet(
void* target,
const String& val);
121 String doGet(
const void* target)
const;
122 void doSet(
void* target,
const String& val);
129 String doGet(
const void* target)
const;
130 void doSet(
void* target,
const String& val);
137 String doGet(
const void* target)
const;
138 void doSet(
void* target,
const String& val);
145 String doGet(
const void* target)
const;
146 void doSet(
void* target,
const String& val);
184 virtual const String&
getType(
void)
const override;
185 virtual void _updateRenderQueue(RenderQueue* queue, Ogre::list<Particle*>::type& currentParticles,
bool cullIndividually)
override;
186 virtual void visitRenderables(Renderable::Visitor* visitor,
bool debugRenderables =
false)
override;
192 virtual void _notifyAttached(Node* parent,
bool isTagPoint =
false)
override;
204 virtual const MaterialPtr&
getMaterial(
void)
const override;
208 virtual const LightList&
getLights(
void)
const override;
218 void addParticle(uint8* pDataVB,
const Particle& particle)
const;
237 const String&
getType()
const override;
238 ParticleSystemRenderer*
createInstance(
const String& name )
override;
249 #endif // __OGRE_SHADER_PARTICLE_RENDERER_H__
virtual const LightList & getLights(void) const override
Real mRadius
maximum distance between particles and parent node
void setVertexFormatTotalTTL(bool bUse)
static CmdVertexFormatTimeFrag msVertexFmtTimeFrag
bool mVertexFormatSize
particle size (width and height - float2)
Node * mParentNode
parent node for particle system - used for world transformation
virtual void _notifyAttached(Node *parent, bool isTagPoint=false) override
Command objects for defining vertex format (see ParamCommand).
bool getVertexFormatTotalTTL() const
Vector2 mDefaultParticleSize
other informations
Vector2 mTexCoordTable[4]
default texture coordinates
static CmdVertexFormatTotalTTL msVertexFmtTotalTTL
virtual void _notifyParticleResized(void) override
void setVertexFormatTimeFragment(bool bUse)
bool mKeepInLocalSpace
control transformation matrix for particles
virtual void _notifyCurrentCamera(Camera *cam) override
void destroyInstance(ParticleSystemRenderer *inst) override
void setVertexFormatTimeFragmentInv(bool bUse)
static CmdVertexFormatRotation msVertexFmtRotation
MaterialPtr mMaterial
rendering data
virtual void _notifyParticleQuota(size_t quota) override
virtual void getWorldTransforms(Matrix4 *xform) const override
bool getVertexFormatSize() const
virtual Real getSquaredViewDepth(const Camera *cam) const override
static CmdVertexFormatColour msVertexFmtColour
bool mVertexFormatRotationSpeed
particle rotation speed (radians/s - float1)
virtual SortMode _getSortMode(void) const override
virtual void _updateRenderQueue(RenderQueue *queue, Ogre::list< Particle * >::type ¤tParticles, bool cullIndividually) override
bool getVertexFormatTexture() const
virtual ~ShaderParticleRenderer()
virtual void getRenderOperation(RenderOperation &op) override
void setVertexFormatRotationSpeed(bool bUse)
virtual ParticleVisualData * _createVisualData(void) override
bool mParentIsTagPoint
true if parent node is tag point
virtual const MaterialPtr & getMaterial(void) const override
Factory class for ShaderParticleRenderer.
LightList mLightList
light list for renderable
virtual void setRenderQueueGroup(uint8 queueID) override
bool getVertexFormatTimeFragmentInv() const
static CmdVertexFormatDirection msVertexFmtDirection
void setVertexFormatTTL(bool bUse)
bool allocateBuffers(size_t iNumParticles)
allocate hardware buffers and prepare them for filling particles
Specialisation of ParticleSystemRenderer to render particles using a custom shaders.
bool mVertexFormatTotalTTL
particle total ttl (float1)
bool getVertexFormatTimeFragment() const
bool getVertexFormatDirection() const
bool getVertexFormatRotationSpeed() const
virtual void _notifyParticleRotated(void) override
bool mVertexFormatRotation
particle rotation (radians - float1)
virtual const String & getType(void) const override
bool mVertexFormatTexture
true if particles use texture (float2)
static CmdVertexFormatTimeFragInv msVertexFmtTimeFragInv
void setRenderQueueGroupAndPriority(Ogre::uint8, Ogre::ushort)
ParticleSystemRenderer * createInstance(const String &name) override
bool mVertexFormatTTL
particle ttl (float1)
void setVertexFormatTexture(bool bUse)
bool getVertexFormatRotation() const
virtual void _destroyVisualData(ParticleVisualData *vis) override
void setVertexFormatSize(bool bUse)
bool getVertexFormatTTL() const
SortMode mSortMode
particle sorting
void addParticle(uint8 *pDataVB, const Particle &particle) const
add particle to vertex buffer
void setVertexFormatDirection(bool bUse)
void setVertexFormatColour(bool bUse)
virtual void _notifyDefaultDimensions(Real width, Real height) override
bool mVertexFormatDirection
particle direction (float3)
static CmdVertexFormatSize msVertexFmtSize
const String rendererTypeName
static CmdVertexFormatTexture msVertexFmtTexture
static CmdVertexFormatTTL msVertexFmtTTL
bool mVertexFormatTimeFragmentInv
particle inverse time fragment (1.0f - ttl / total ttl) (float1) (value 0.0f - 1.0f)
const String rendererTypeName
virtual void visitRenderables(Renderable::Visitor *visitor, bool debugRenderables=false) override
const String & getType() const override
void setVertexFormatRotation(bool bUse)
virtual void _setMaterial(MaterialPtr &mat) override
ulong mLightListUpdated
indicator if we need update light list
static CmdVertexFormatRotationSpeed msVertexFmtRotationSpeed
bool mVertexFormatTimeFragment
particle time fragment (ttl / total ttl) (float1) (value 0.0f - 1.0f)
bool getVertexFormatColour() const
virtual void setKeepParticlesInLocalSpace(bool keepLocal) override
bool mVertexFormatColour
vertex format for particles