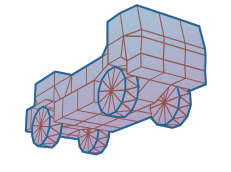 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #include <OgreMatrix3.h>
25 #include <OgreVector3.h>
123 return {result[0], result[1], (1.f - result[0] - result[1]), result[2]};
135 m_matrix = Ogre::Matrix3{ u[0], v[0], n[0],
137 u[2], v[2], n[2] }.Inverse();
Represents a triangle in three-dimensional space.
Ogre::Vector3 normal() const
Return normal vector of the triangle.
const Ogre::Vector3 v
Span vector v.
const Ogre::Vector3 u
Span vector u.
const Ogre::Vector3 c
Vertex c.